题目
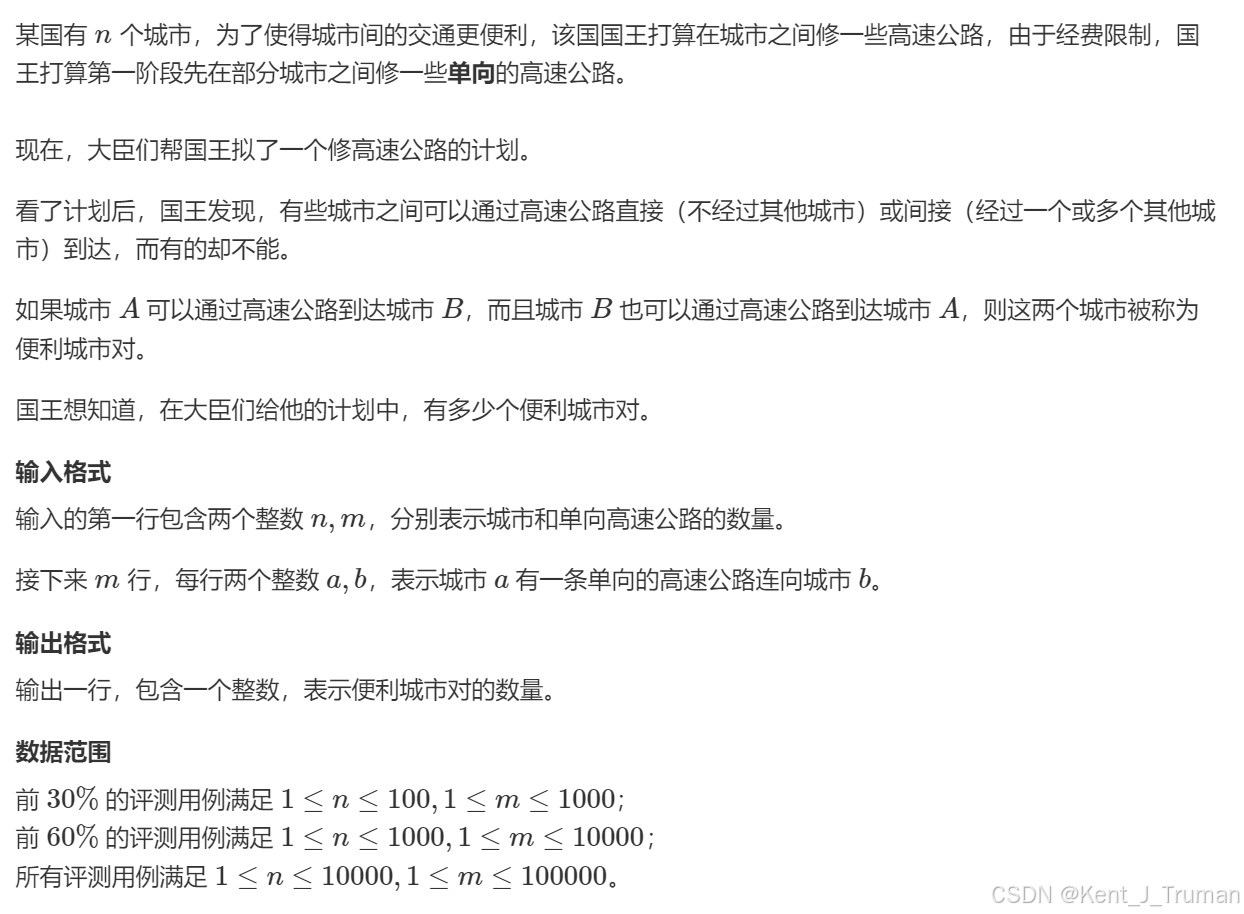
BFS暴力代码 60p
#include <bits/stdc++.h>
using namespace std;
const int N = 1010;
const int M = 10010;
int g[N][N];
int n, m;
int h[N], e[M], ne[M], idx;
void add(int a, int b) // 添加一条边a->b
{
e[idx] = b, ne[idx] = h[a], h[a] = idx ++ ;
}
void bfs(int u)
{
queue<int> q;
q.push(u);
while(q.size())
{
int t = q.front(); q.pop();
for(int i = h[t]; ~i; i = ne[i])
{
int j = e[i];
if(g[u][j]) continue;
g[u][j] = 1;
q.push(j);
}
}
}
int main()
{
memset(h, -1, sizeof h);
scanf("%d%d", &n, &m);
for(int i = 1; i <= m; i++)
{
int a, b;
scanf("%d%d", &a, &b);
add(a, b);
}
for(int i = 1; i <= n; i++)
bfs(i);
int ans = 0;
for(int i = 1; i <= n; i++)
for(int j = i+1; j <= n; j++)
if(g[i][j] && g[j][i]) ans++;
cout << ans;
}
Tarjan代码
#include <bits/stdc++.h>
using namespace std;
using ll = long long;
const int N = 1e4+10;
const int M = 1e5+10;
int h[N], e[M], ne[M], idx; //链式前向星
int dfn[N], low[N], tot; //时间戳 追溯值 分配器
int scc[N], sz[N], cnt; //强连通分量归属 大小 分配器
int stk[N], tt; //栈相关
bool in[N];
int n, m;
void add(int a, int b) // 添加一条边a->b
{
e[idx] = b, ne[idx] = h[a], h[a] = idx ++ ;
}
void tarjan(int u)
{
dfn[u] = ++tot, low[u] = tot;
stk[++tt] = u; in[u] = 1;
for(int i = h[u]; ~i; i = ne[i])
{
int j = e[i];
if(!dfn[j]) //未访问过,先递归,再更新追溯值
{
tarjan(j);
low[u] = min(low[u], low[j]);
}
else if(in[j]) //儿子访问过,但仍在栈中,说明成环,拿时间戳
{
low[u] = min(low[u], dfn[j]);
}
}
if(dfn[u] == low[u]) //时间戳与追溯值一致,x为强连通分量的根节点,开始退栈
{
int t; ++cnt;
do
{
t = stk[tt--]; in[t] = 0;
scc[t] = cnt;
sz[cnt]++;
}while(t != u);
}
}
int main()
{
memset(h, -1, sizeof h);
scanf("%d%d", &n, &m);
for(int i = 1; i <= m; i++)
{
int a, b;
scanf("%d%d", &a, &b);
add(a, b);
}
for(int i = 1; i <= n; i++)
if(!dfn[i]) tarjan(i);
ll ans = 0;
for(int i = 1; i <= cnt; i++)
ans += 1ll * sz[i] * (sz[i] - 1) / 2;
cout << ans;
}